How to Connect LCD to Arduino
The LCD (Liquid Crystal Display) is a commonly used display for Arduino projects, as it provides a simple way to output information to the user such as text and basic characters. It is a useful display for beginners and experienced users alike and is typically one of the first displays people use when they start using an Arduino board.
This tutorial will show you how to connect and interact between a 16x2 LCD Character Display and an Arduino UNO board using serial communication.
The principles in this tutorial can be applied to other LCD displays and other development boards as well. Let's get started!
LCD - Arduino Tutorial - Table of Contents
- Hardware and tools needed
- LCD pinout
- Arduino pinout
- Circuit - connection diagram and schematic for SPI communication
- Example code using SPI interface
- Circuit - connection diagram and schematic for RS232 TTL communication
- Hello World! example code using RS232 TTL interface
- Troubleshooting Your LCD - Arduino Project
- Additional resources
Hardware and Tools Needed
LCDs come in various sizes and configurations, but the most commonly used is the 16x2 LCD. It has 2 rows that can fit 16 characters per row.
We will be using the 16x2 Newhaven display part number NHD-0216K3Z-NSW-BBW-V3, which has a built-in PIC16F690 microcontroller. This LCD supports three serial interfaces: I2C, SPI, and RS-232 (TTL). This article will focus on the SPI and RS-232 interfaces and provide code examples for both.
This display is available for purchase here. The datasheet and product specs can be viewed or downloaded here.
Please note that using the SPI interface requires soldering a resistor to the display, whereas the RS-232 TTL interface does not. Therefore, if you prefer to follow this tutorial without soldering the resistor, you can follow the RS-232 TTL interface instructions and code example section.
Things you will need to connect Arduino to an LCD
- LCD 16x2
- Single row pin header connector
- Arduino UNO
- breadboard
- Jumper wires
- Solder and soldering iron
- USB A male to B Male Cable (printer cable)
- Arduino IDE
- 0 ohm resistor (optional - for SPI communication only)
We suggest reading our blog article How to Protect Electronics from ESD before diving into this tutorial, especially If you are a beginner with electronics.
16x2 LCD Pinout
The Newhaven 16x2 character LCD offers a simple way to interact with the Arduino UNO board using serial communication. The pins on the P1 port are specifically designed for RS232 TTL serial communication, and the pins on the P2 port are for serial I2C and SPI communication.
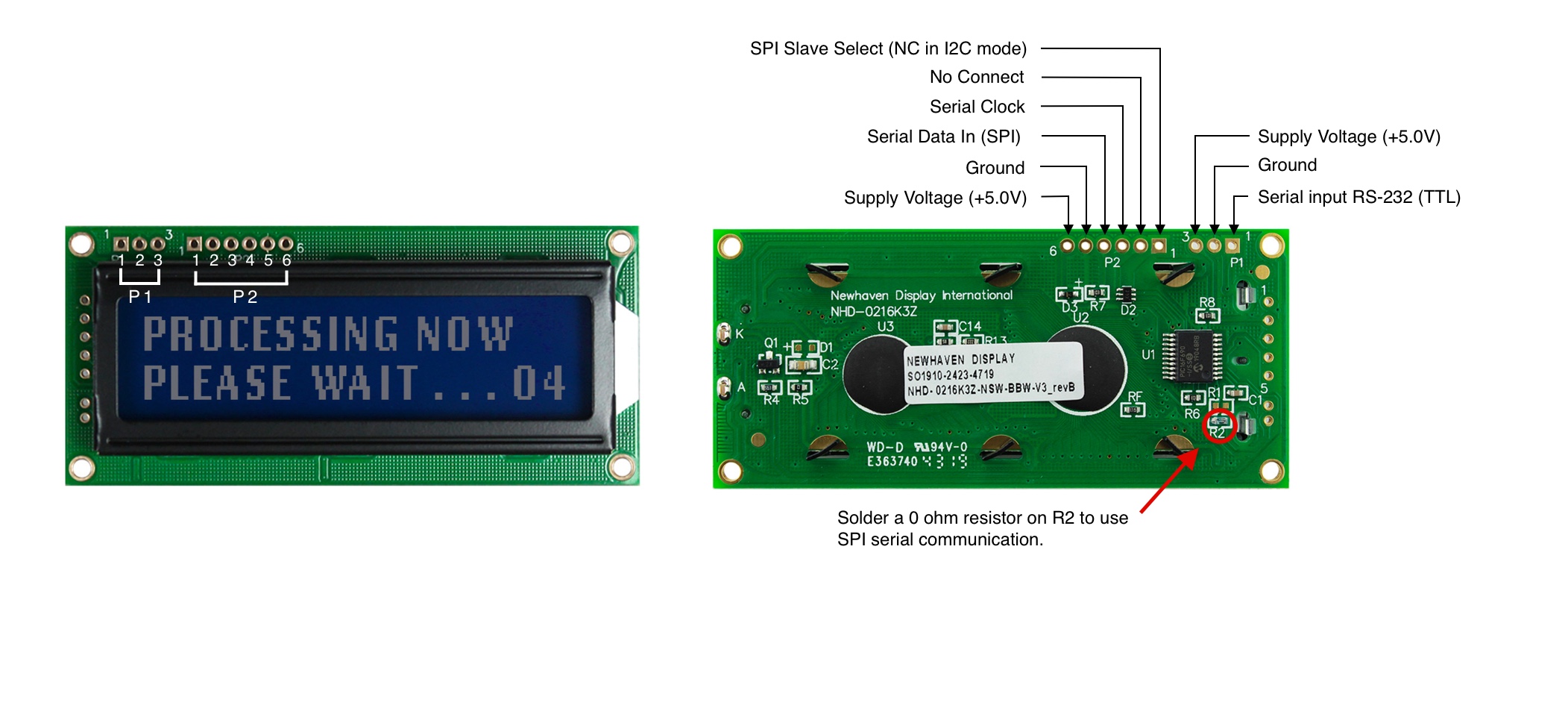
P1 Pin Description - RS232 TTL Communication
Pin No. | Symbol | Function Description |
---|---|---|
1 | RX | RS-232 (TTL) Serial input |
2 | V SS | Ground |
3 | V DD | Supply Voltage (+5.0V) |
P2 Pin Description - SPI and I2C Communication
Pin No. | Symbol | Function Description |
---|---|---|
1 | SPISS | SPI Slave Select (NC in I2C mode) |
2 | SDO | No Connect |
3 | SCK/SCL | Serial Clock |
4 | SDI/SDA | Serial Data In (SPI) / Serial Data (I2C) |
5 | V SS | Ground |
6 | V DD | Supply Voltage (+5.0V) |
The complete LCD pinout specification can be viewed here.
Arduino Pinout
The Arduino UNO board has 20 input/output pins, including 14 digital pins and 6 analog pins, and for this project, we will use the 5V and GND pins from the power signals, pins 10, 11, and 12 for SPI or I2C, and pin 7 for RS232 TTL.
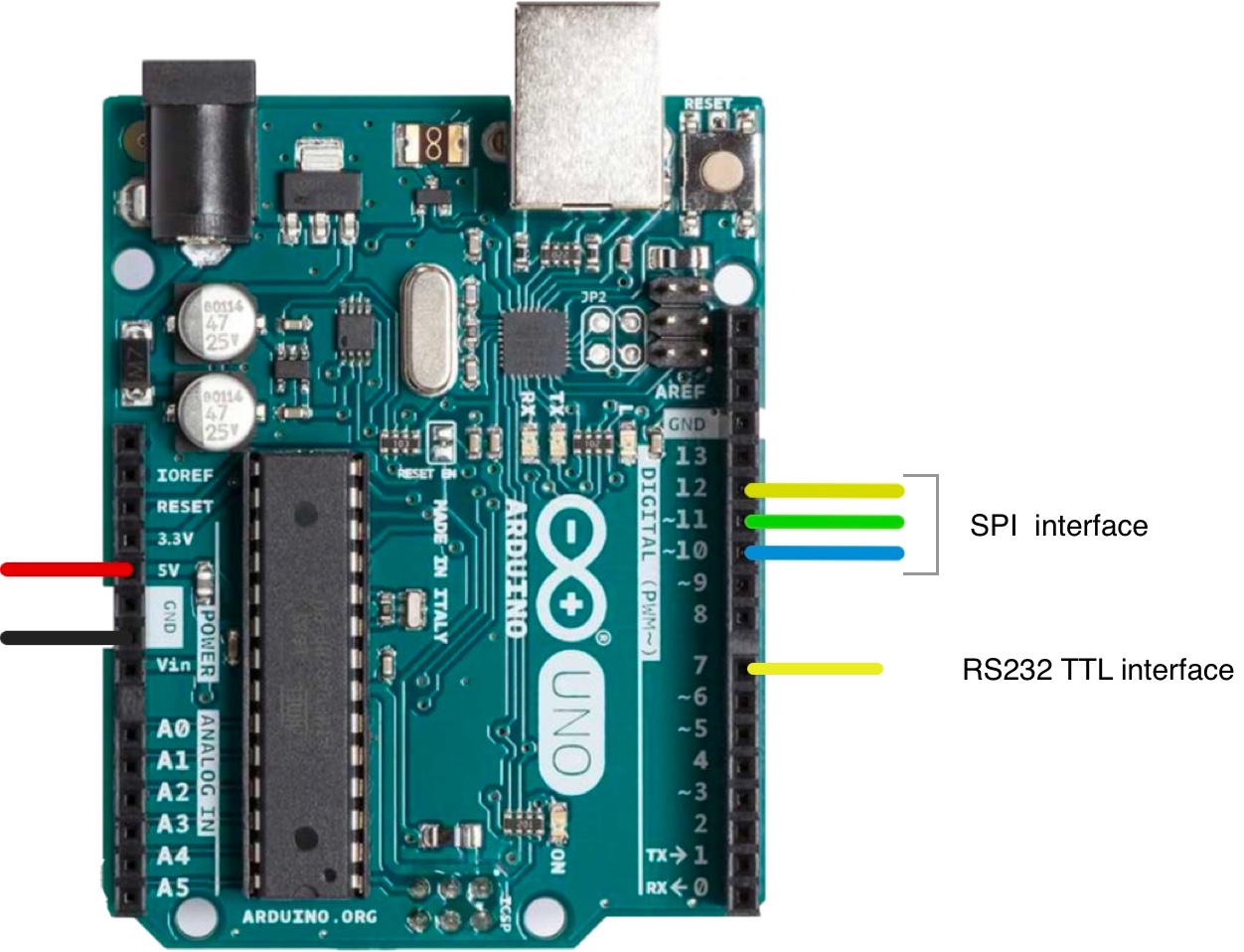
The complete Arduino UNO pinout schematic can be viewed here.
Circuit - Connection Diagram and Schematic for SPI Communication
The Serial Peripheral Interface (SPI) is a synchronous serial communication bus used for short-distance, high-speed communication between multiple devices. The connection circuit for SPI communication between the Arduino (master device) and the LCD (slave device) uses five wires to communicate as described in the table below:
Arduino | LCD | Connection Type |
---|---|---|
5V Pin | Pin 6: V DD | Power |
Ground Pin | Pin 5: Ground | Ground |
Pin 10: Slave Select | Pin 3: SCK/SCL | Serial Clock |
Pin 11: MISO | Pin 4: Serial Data In (SPI) / Serial Data (I2C) | Serial Data In |
Pin 12: MOSI | Pin 1: SPISS | SPI Slave Select |
Note:
Enabling SPI requires R1 to be open and R2 to be shortened as specified in the
datasheet. Also, before starting, ensure the Arduino is powered off while wiring up the circuit.
SPI Wiring circuit
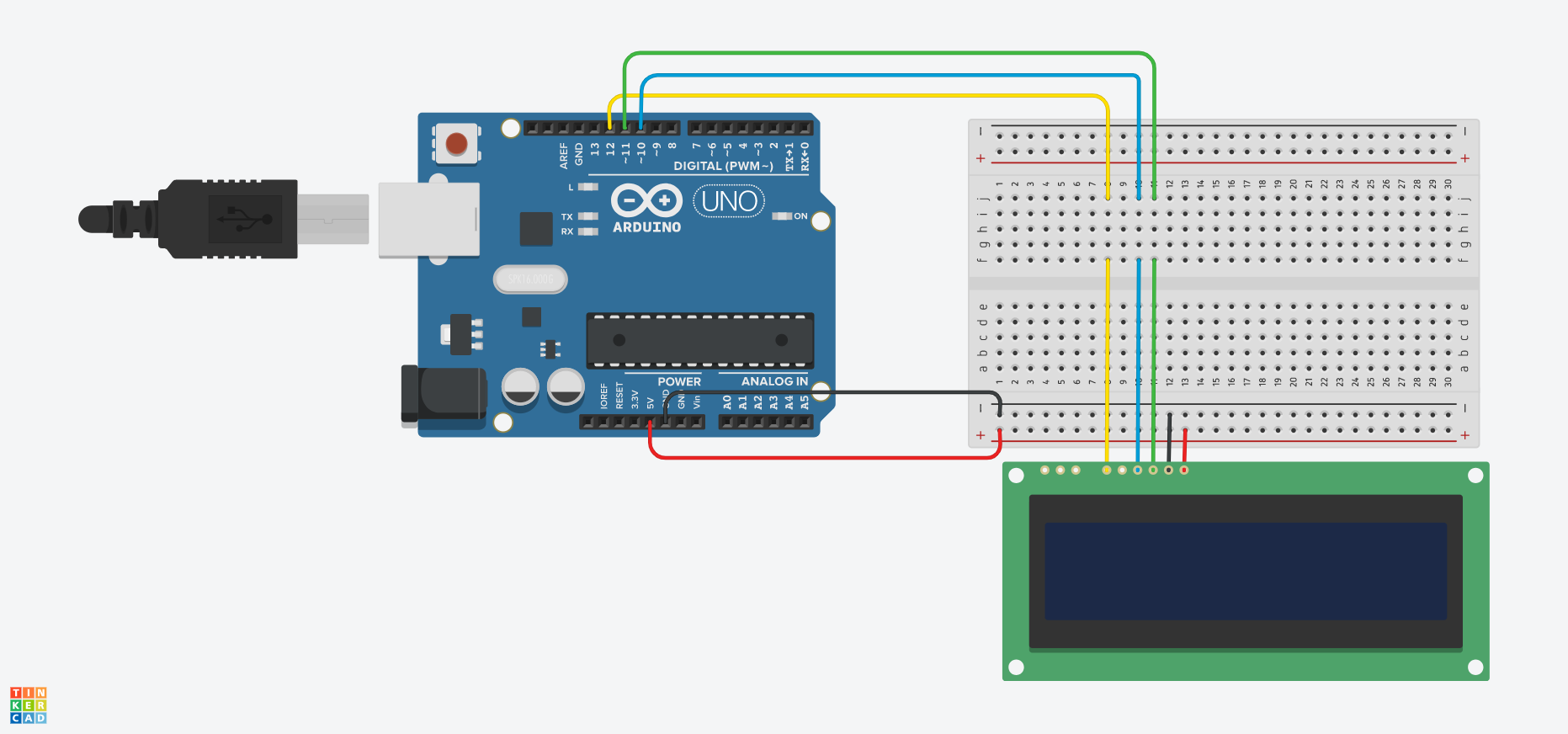
SPI Wiring Schematic
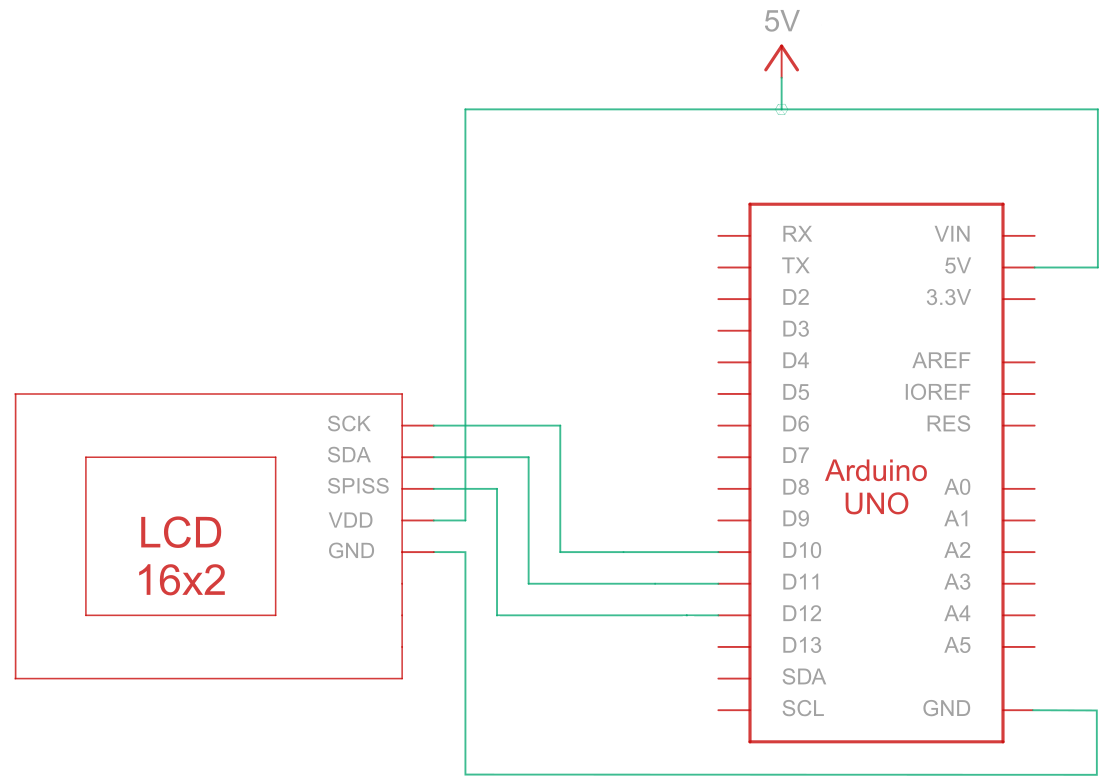
Arduino - LCD Example Code Using SPI interface
The following code examples demonstrate how to use serial communication between Arduino and a 16x2 character LCD using the SPI interface. You will find helpful comments in the code examples, but let's break down some of the functions below:
- The
SPI_Out()
function sends commands and data to the LCD display through SPI. It does this by iterating through the 8 bits of the input byte and sending each bit's value to the LCD display. - The
Set_Pins()
function configures the SPI pins as output pins. - The
Set_Contrast()
function sets the contrast of the LCD display. - The
Set_Backlight()
function sets the backlight of the LCD display. - The
Clear_Display()
function clears the LCD screen. - The
Set_Cursor()
function enables or disables the blinking cursor on the LCD display, depending on the input parameter. - The
setup()
function is the main function and is executed once when the Arduino starts. It is used to initialize the settings, variables and any run any other necessary functions and configurations for the project. - The
loop()
function runs in a continuous loop for the entire duration that the Arduino board is powered on. In some examples below, this function is empty and does nothing because we just need to run some functions once.
Arduino - LCD code examples using SPI:
- Hello world!
- Display text on the first and second row
- Display long text
- Display text with a blinking cursor
- Cursor + autoscroll text
- Autoscroll
- Contrast
- Backlight
- Characters/font tables
You can adapt and expand the code examples to suit your specific Arduino-based project requirements.
Hello world!
The following code example displays "Hello Word!" on the LCD.
/* SPI Wiring Reference for: NHD-0216K3Z-NSW-BBW-V3 --------------------------- LCD | Arduino --------------------------- 1(SPISS) --> 12 2(SDO) --> No Connect 3(SCK) --> 10 4(SDA) --> 11 5(VSS) --> Ground 6(VDD) --> 5V */ /***************************************************** Arduino pin definition /*****************************************************/ #define SPISS_PIN 12 #define SDA_PIN 11 #define SCK_PIN 10 /***************************************************** SPI function to send command and data. For more information on SPI please visit our Support Center /*****************************************************/ void SPI_Out(unsigned char a) { digitalWrite(SPISS_PIN, LOW); for (int n = 0; n < 8; n++) { if ((a & 0x80) == 0x80) { digitalWrite(SDA_PIN, HIGH); delayMicroseconds(200); } else { digitalWrite(SDA_PIN, LOW); } delayMicroseconds(200); a = a << 1; digitalWrite(SCK_PIN, LOW); digitalWrite(SCK_PIN, HIGH); delayMicroseconds(200); } digitalWrite(SCK_PIN, HIGH); delayMicroseconds(200); digitalWrite(SPISS_PIN, HIGH); } /***************************************************** Set Arduino UNO IO ports as Output mode /*****************************************************/ void Set_Pins() { pinMode(SPISS_PIN, OUTPUT); pinMode(SCK_PIN, OUTPUT); pinMode(SDA_PIN, OUTPUT); } /***************************************************** Set LCD Contrast /*****************************************************/ void Set_Contrast() { SPI_Out(0xFE); //prefix command SPI_Out(0x52); //contrast command SPI_Out(0x28); //set contrast value delayMicroseconds(500); } /***************************************************** Set LCD Backlight /*****************************************************/ void Set_Backlight() { SPI_Out(0xFE); //prefix command SPI_Out(0x53); //backlight command SPI_Out(0x08); //set backlight value delayMicroseconds(100); } /***************************************************** Clear LCD /*****************************************************/ void Clear_Display() { SPI_Out(0xFE); //prefix command SPI_Out(0x51); //clear display command delayMicroseconds(1500); } /***************************************************** Set cursor Mode = 0 sets Blinking cursor off Mode = 1 sets Blinking cursor on /*****************************************************/ void Set_Cursor(int mode) { SPI_Out(0xfe); //prefix command if (mode == 0) { SPI_Out(0x4c); //Blinking cursor off } else if (mode == 1) { SPI_Out(0x4b); //Blinking cursor on } delayMicroseconds(100); } /***************************************************** Write "Hello World!" to LCD /*****************************************************/ void Hello_World() { SPI_Out(0x48); // H SPI_Out(0x65); // E SPI_Out(0x6C); // l SPI_Out(0x6C); // l SPI_Out(0x6F); // o SPI_Out(0x11); // Blank SPI_Out(0x57); // W SPI_Out(0x6f); // o SPI_Out(0x72); // r SPI_Out(0x6c); // l SPI_Out(0x64); // d SPI_Out(0x21); // ! } /***************************************************** Main function /*****************************************************/ void setup() { Set_Pins(); //set IO ports Set_Cursor(0); //turns off blinking cursor Set_Contrast(); //set display contrast Set_Backlight(); //set display backlight Clear_Display(); //clear display Hello_World(); //display "Hello World!" } void loop() { }
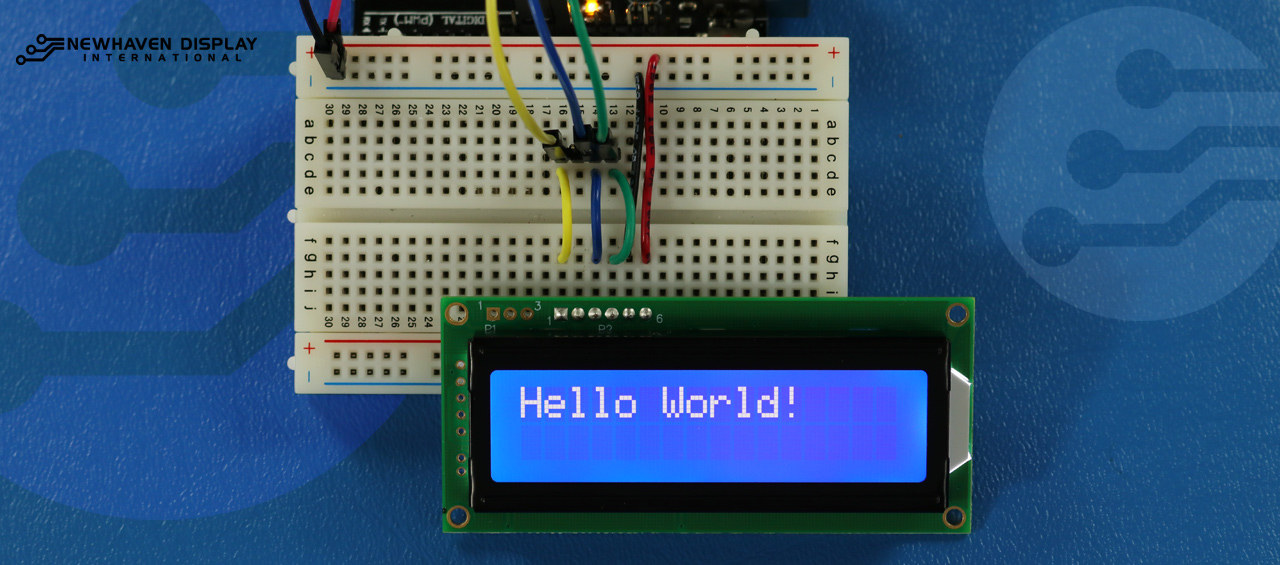
Display text on the first and second row
This code will show the text "First Line" in the first row and "Second Line" in the second row.
/***************************************************** This code was written for the Arduino UNO using SPI Interface. This code displays text in row one and row two of LCD. /* SPI Wiring Reference for: NHD-0216K3Z-NSW-BBW-V3 --------------------------- LCD | Arduino --------------------------- 1(SPISS) --> 12 2(SDO) --> No Connect 3(SCK) --> 10 4(SDA) --> 11 5(VSS) --> Ground 6(VDD) --> 5V */ /***************************************************** Arduino pin definition /*****************************************************/ #define SPISS_PIN 12 #define SDA_PIN 11 #define SCK_PIN 10 /***************************************************** SPI function to send command and data. For more information on SPI please visit our Support Center /*****************************************************/ void SPI_Out(unsigned char a) { digitalWrite(SPISS_PIN, LOW); for (int n = 0; n < 8; n++) { if ((a & 0x80) == 0x80) { digitalWrite(SDA_PIN, HIGH); delayMicroseconds(200); } else { digitalWrite(SDA_PIN, LOW); } delayMicroseconds(200); a = a << 1; digitalWrite(SCK_PIN, LOW); digitalWrite(SCK_PIN, HIGH); delayMicroseconds(200); } digitalWrite(SCK_PIN, HIGH); delayMicroseconds(200); digitalWrite(SPISS_PIN, HIGH); } /***************************************************** Set Arduino UNO IO ports as Output mode /*****************************************************/ void Set_Pins() { pinMode(SPISS_PIN, OUTPUT); pinMode(SCK_PIN, OUTPUT); pinMode(SDA_PIN, OUTPUT); } /***************************************************** Set LCD Contrast /*****************************************************/ void Set_Contrast() { SPI_Out(0xFE); //prefix command SPI_Out(0x52); //contrast command SPI_Out(0x28); //set contrast value delayMicroseconds(500); } /***************************************************** Set LCD Backlight /*****************************************************/ void Set_Backlight() { SPI_Out(0xFE); //prefix command SPI_Out(0x53); //backlight command SPI_Out(0x08); //set backlight value delayMicroseconds(100); } /***************************************************** Clear LCD /*****************************************************/ void Clear_Display() { SPI_Out(0xFE); //prefix command SPI_Out(0x51); //clear display command delayMicroseconds(1500); } /***************************************************** Write "First Line" to row one on LCD /*****************************************************/ void Send_First_Line_Text() { SPI_Out(0x46); // F SPI_Out(0x69); // i SPI_Out(0x72); // r SPI_Out(0x73); // s SPI_Out(0x74); // t SPI_Out(0x20); // blank space SPI_Out(0x6c); // l SPI_Out(0x69); // i SPI_Out(0x6e); // n SPI_Out(0x65); // e } /***************************************************** Write "Second Line" to row two on LCD /*****************************************************/ void Send_Second_Line_Text() { SPI_Out(0x53); // S SPI_Out(0x65); // e SPI_Out(0x63); // c SPI_Out(0x6f); // o SPI_Out(0x6e); // n SPI_Out(0x64); // d SPI_Out(0x20); // blank space SPI_Out(0x6c); // l SPI_Out(0x69); // i SPI_Out(0x6e); // n SPI_Out(0x65); // e } /***************************************************** Set Row address Line = 1 sets for first row Line = 2 sets for second row /*****************************************************/ void Set_Row(int line) { SPI_Out(0xfe); //prefix command SPI_Out(0x45); //set cursor command if (line == 1) { SPI_Out(0x00); //cursor position: row one, column one } else if (line == 2) { SPI_Out(0x40); //cursor position: row two, column one } delayMicroseconds(100); } /***************************************************** Main function /*****************************************************/ void setup() { Set_Pins(); //set IO ports Set_Contrast(); //set display contrast Set_Backlight(); //set display backlight Clear_Display(); //clear display Set_Row(1); Send_First_Line_Text(); //writes to first line of display Set_Row(2); Send_Second_Line_Text(); //writes to second line of display delay(1000); } void loop() { }
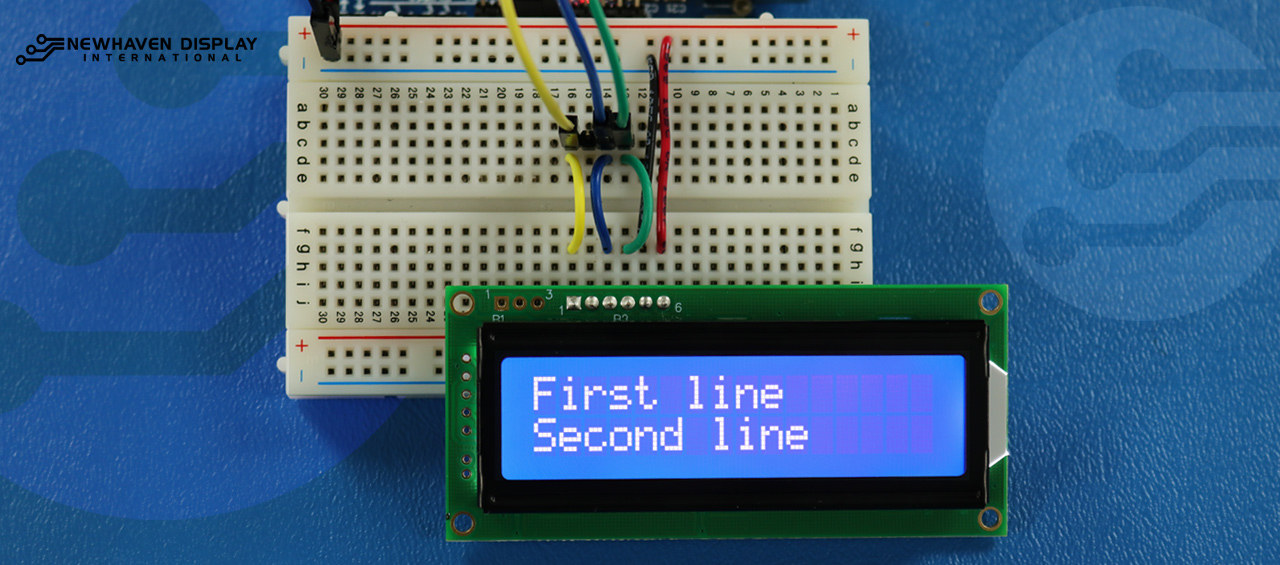
Display long text
This code displays text that extends to the second row. For this example, we will display the text "NHD-0216K3Z-NSW-BBW-V3".
/***************************************************** This code displays the part number: NHD-0216K3Z-NSW-BBW-V3. /* SPI Wiring Reference for: NHD-0216K3Z-NSW-BBW-V3 --------------------------- LCD | Arduino --------------------------- 1(SPISS) --> 12 2(SDO) --> No Connect 3(SCK) --> 10 4(SDA) --> 11 5(VSS) --> Ground 6(VDD) --> 5V */ /***************************************************** Arduino pin definition /*****************************************************/ #define SPISS_PIN 12 #define SDA_PIN 11 #define SCK_PIN 10 /***************************************************** SPI function to send command and data. For more information on SPI please visit our Support Center /*****************************************************/ void SPI_Out(unsigned char a) { digitalWrite(SPISS_PIN, LOW); for (int n = 0; n < 8; n++) { if ((a & 0x80) == 0x80) { digitalWrite(SDA_PIN, HIGH); delayMicroseconds(200); } else { digitalWrite(SDA_PIN, LOW); } delayMicroseconds(200); a = a << 1; digitalWrite(SCK_PIN, LOW); digitalWrite(SCK_PIN, HIGH); delayMicroseconds(200); } digitalWrite(SCK_PIN, HIGH); delayMicroseconds(200); digitalWrite(SPISS_PIN, HIGH); } /***************************************************** Set Arduino UNO IO ports as Output mode /*****************************************************/ void Set_Pins() { pinMode(SPISS_PIN, OUTPUT); pinMode(SCK_PIN, OUTPUT); pinMode(SDA_PIN, OUTPUT); } /***************************************************** Set LCD Contrast /*****************************************************/ void Set_Contrast() { SPI_Out(0xFE); //prefix command SPI_Out(0x52); //contrast command SPI_Out(0x28); //set contrast value delayMicroseconds(500); } /***************************************************** Set LCD Backlight /*****************************************************/ void Set_Backlight() { SPI_Out(0xFE); //prefix command SPI_Out(0x53); //backlight command SPI_Out(0x08); //set backlight value delayMicroseconds(100); } /***************************************************** Clear LCD /*****************************************************/ void Clear_Display() { SPI_Out(0xFE); //prefix command SPI_Out(0x51); //clear display command delayMicroseconds(1500); } /***************************************************** Set cursor Mode = 0 sets Blinking cursor off Mode = 1 sets Blinking cursor on /*****************************************************/ void Set_Cursor(int mode) { SPI_Out(0xfe); //prefix command if (mode == 0) { SPI_Out(0x4c); //Blinking cursor off } else if (mode == 1) { SPI_Out(0x4b); //Blinking cursor on } delayMicroseconds(100); } /***************************************************** Set Row address Line = 1 sets for first row Line = 2 sets for second row /*****************************************************/ void Set_Row(int line) { SPI_Out(0xfe); //prefix command SPI_Out(0x45); //set cursor command if (line == 1) { SPI_Out(0x00); //cursor position: row one, column one } else if (line == 2) { SPI_Out(0x40); //cursor position: row two, column one } delayMicroseconds(100); } /***************************************************** Write "NHD-0216K3Z-NSW-BBW-V3" to LCD /*****************************************************/ void Part_Number() { Set_Row(1); SPI_Out(0x4e); // N SPI_Out(0x48); // H SPI_Out(0x44); // D SPI_Out(0xb0); // - SPI_Out(0x30); // 0 SPI_Out(0x32); // 2 SPI_Out(0x31); // 1 SPI_Out(0x36); // 6 SPI_Out(0x4b); // K SPI_Out(0x33); // 3 SPI_Out(0x5a); // Z SPI_Out(0xb0); // - SPI_Out(0x4e); // N SPI_Out(0x53); // S SPI_Out(0x57); // W SPI_Out(0xb0); // - Set_Row(2); SPI_Out(0x42); // B SPI_Out(0x42); // B SPI_Out(0x57); // W SPI_Out(0xb0); // - SPI_Out(0x56); // V SPI_Out(0x33); // 3 } /***************************************************** Main function /*****************************************************/ void setup() { Set_Pins(); //set IO ports Set_Cursor(0); //turns off blinking cursor Set_Contrast(); //set display contrast Set_Backlight(); //set display backlight Clear_Display(); //clear display Part_Number(); //display "NHD-0216K3Z-NSW-BBW-V3" } void loop() { }
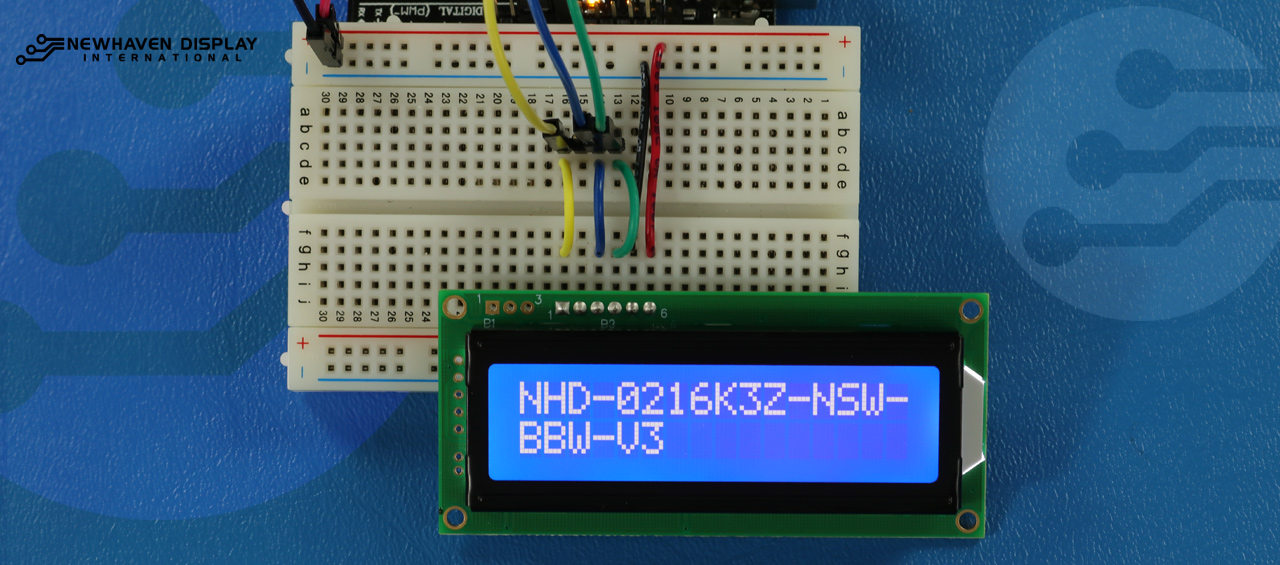
Blink - Display text with blinking cursor
This code displays text with a blinking cursor. For this example, we will display the text "NHD-0216K3Z-NSW-BBW-V3".
/***************************************************** This code displays the part number: NHD-0216K3Z-NSW-BBW-V3 with cursor on. /* SPI Wiring Reference for: NHD-0216K3Z-NSW-BBW-V3 --------------------------- LCD | Arduino --------------------------- 1(SPISS) --> 12 2(SDO) --> No Connect 3(SCK) --> 10 4(SDA) --> 11 5(VSS) --> Ground 6(VDD) --> 5V */ /***************************************************** Arduino pin definition /*****************************************************/ #define SPISS_PIN 12 #define SDA_PIN 11 #define SCK_PIN 10 /***************************************************** SPI function to send command and data. For more information on SPI please visit our Support Center /*****************************************************/ void SPI_Out(unsigned char a) { digitalWrite(SPISS_PIN, LOW); for (int n = 0; n < 8; n++) { if ((a & 0x80) == 0x80) { digitalWrite(SDA_PIN, HIGH); delayMicroseconds(200); } else { digitalWrite(SDA_PIN, LOW); } delayMicroseconds(200); a = a << 1; digitalWrite(SCK_PIN, LOW); digitalWrite(SCK_PIN, HIGH); delayMicroseconds(200); } digitalWrite(SCK_PIN, HIGH); delayMicroseconds(200); digitalWrite(SPISS_PIN, HIGH); } /***************************************************** Set Arduino UNO IO ports as Output mode /*****************************************************/ void Set_Pins() { pinMode(SPISS_PIN, OUTPUT); pinMode(SCK_PIN, OUTPUT); pinMode(SDA_PIN, OUTPUT); } /***************************************************** Set LCD Contrast /*****************************************************/ void Set_Contrast() { SPI_Out(0xFE); //prefix command SPI_Out(0x52); //contrast command SPI_Out(0x28); //set contrast value delayMicroseconds(500); } /***************************************************** Set LCD Backlight /*****************************************************/ void Set_Backlight() { SPI_Out(0xFE); //prefix command SPI_Out(0x53); //backlight command SPI_Out(0x08); //set backlight value delayMicroseconds(100); } /***************************************************** Clear LCD /*****************************************************/ void Clear_Display() { SPI_Out(0xFE); //prefix command SPI_Out(0x51); //clear display command delayMicroseconds(1500); } /***************************************************** Set cursor Mode = 0 sets Blinking cursor off Mode = 1 sets Blinking cursor on /*****************************************************/ void Set_Cursor(int mode) { SPI_Out(0xfe); //prefix command if (mode == 0) { SPI_Out(0x4c); //Blinking cursor off } else if (mode == 1) { SPI_Out(0x4b); //Blinking cursor on } delayMicroseconds(100); } /***************************************************** Set Row address Line = 1 sets for first row Line = 2 sets for second row /*****************************************************/ void Set_Row(int line) { SPI_Out(0xfe); //prefix command SPI_Out(0x45); //set cursor command if (line == 1) { SPI_Out(0x00); //cursor position: row one, column one } else if (line == 2) { SPI_Out(0x40); //cursor position: row two, column one } delayMicroseconds(100); } /***************************************************** Write "NHD-0216K3Z-NSW-BBW-V3" to LCD delay(500) = 500 Milliseconds /*****************************************************/ void Part_Number() { Set_Row(1); SPI_Out(0x4e); // N delay(500); SPI_Out(0x48); // H delay(500); SPI_Out(0x44); // D delay(500); SPI_Out(0xb0); // - delay(500); SPI_Out(0x30); // 0 delay(500); SPI_Out(0x32); // 2 delay(500); SPI_Out(0x31); // 1 delay(500); SPI_Out(0x36); // 6 delay(500); SPI_Out(0x4b); // K delay(500); SPI_Out(0x33); // 3 delay(500); SPI_Out(0x5a); // Z delay(500); SPI_Out(0xb0); // - delay(500); SPI_Out(0x4e); // N delay(500); SPI_Out(0x53); // S delay(500); SPI_Out(0x57); // W delay(500); SPI_Out(0xb0); // - Set_Row(2); delay(500); SPI_Out(0x42); // B delay(500); SPI_Out(0x42); // B delay(500); SPI_Out(0x57); // W delay(500); SPI_Out(0xb0); // - delay(500); SPI_Out(0x56); // V delay(500); SPI_Out(0x33); // 3 } /***************************************************** Main function /*****************************************************/ void setup() { Set_Pins(); //set IO ports Set_Contrast(); //set display contrast Set_Backlight(); //set display backlight Clear_Display(); //clear display Set_Cursor(1); //turns on blinking cursor Part_Number(); //display "NHD-0216K3Z-NSW-BBW-V3" } void loop() { }
Cursor + autoscroll text
This code first displays text with the cursor on and then initializes autoscrolling to the right.
/***************************************************** This code was written for the Arduino UNO using SPI Interface. This code displays the part number: NHD-0216K3Z-NSW-BBW-V3 while shifting to the right with a blinking cursor. /* SPI Wiring Reference for: NHD-0216K3Z-NSW-BBW-V3 --------------------------- LCD | Arduino --------------------------- 1(SPISS) --> 12 2(SDO) --> No Connect 3(SCK) --> 10 4(SDA) --> 11 5(VSS) --> Ground 6(VDD) --> 5V */ /***************************************************** Arduino pin definition /*****************************************************/ #define SPISS_PIN 12 #define SDA_PIN 11 #define SCK_PIN 10 /***************************************************** SPI function to send command and data. For more information on SPI please visit our Support Center /*****************************************************/ void SPI_Out(unsigned char a) { digitalWrite(SPISS_PIN, LOW); for (int n = 0; n < 8; n++) { if ((a & 0x80) == 0x80) { digitalWrite(SDA_PIN, HIGH); delayMicroseconds(200); } else { digitalWrite(SDA_PIN, LOW); } delayMicroseconds(200); a = a << 1; digitalWrite(SCK_PIN, LOW); digitalWrite(SCK_PIN, HIGH); delayMicroseconds(200); } digitalWrite(SCK_PIN, HIGH); delayMicroseconds(200); digitalWrite(SPISS_PIN, HIGH); } /***************************************************** Set Arduino UNO IO ports as Output mode /*****************************************************/ void Set_Pins() { pinMode(SPISS_PIN, OUTPUT); pinMode(SCK_PIN, OUTPUT); pinMode(SDA_PIN, OUTPUT); } /***************************************************** Set LCD Contrast /*****************************************************/ void Set_Contrast() { SPI_Out(0xFE); //prefix command SPI_Out(0x52); //contrast command SPI_Out(0x28); //set contrast value delayMicroseconds(500); } /***************************************************** Set LCD Backlight /*****************************************************/ void Set_Backlight() { SPI_Out(0xFE); //prefix command SPI_Out(0x53); //backlight command SPI_Out(0x08); //set backlight value delayMicroseconds(100); } /***************************************************** Clear LCD /*****************************************************/ void Clear_Display() { SPI_Out(0xFE); //prefix command SPI_Out(0x51); //clear display command delayMicroseconds(1500); } /***************************************************** Set cursor Mode = 0 sets Blinking cursor off Mode = 1 sets Blinking cursor on /*****************************************************/ void Set_Cursor(int mode) { SPI_Out(0xfe); //prefix command if (mode == 0) { SPI_Out(0x4c); //Blinking cursor off } else if (mode == 1) { SPI_Out(0x4b); //Blinking cursor on } delayMicroseconds(100); } /***************************************************** Set Row address Line = 1 sets for first row Line = 2 sets for second row /*****************************************************/ void Set_Row(int line) { SPI_Out(0xfe); //prefix command SPI_Out(0x45); //set cursor command if (line == 1) { SPI_Out(0x00); //cursor position: row one, column one } else if (line == 2) { SPI_Out(0x40); //cursor position: row two, column one } delayMicroseconds(100); } /***************************************************** Write "NHD-0216K3Z-NSW-BBW-V3" to LCD /*****************************************************/ void Part_Number() { Set_Row(1); SPI_Out(0x4e); // N SPI_Out(0x48); // H SPI_Out(0x44); // D SPI_Out(0xb0); // - SPI_Out(0x30); // 0 SPI_Out(0x32); // 2 SPI_Out(0x31); // 1 SPI_Out(0x36); // 6 SPI_Out(0x4b); // K SPI_Out(0x33); // 3 SPI_Out(0x5a); // Z SPI_Out(0xb0); // - SPI_Out(0x4e); // N SPI_Out(0x53); // S SPI_Out(0x57); // W SPI_Out(0xb0); // - Set_Row(2); SPI_Out(0x42); // B SPI_Out(0x42); // B SPI_Out(0x57); // W SPI_Out(0xb0); // - SPI_Out(0x56); // V SPI_Out(0x33); // 3 } /***************************************************** Set Shifting mode Mode = 0 Move display one place to the left Mode = 1 Move display one place to the right delay(500) = 500 Milliseconds /*****************************************************/ void Set_Shift(int mode) { SPI_Out(0xfe); //prefix command if (mode == 0) { SPI_Out(0x55); //Move display one place to the left } else if (mode == 1) { SPI_Out(0x56); //Move display one place to the right } delay(500); } /***************************************************** Main function /*****************************************************/ void setup() { Set_Pins(); //set IO ports Set_Contrast(); //set display contrast Set_Backlight(); //set display backlight Clear_Display(); //clear display Set_Cursor(1); //turns on blinking cursor Part_Number(); //display "NHD-0216K3Z-NSW-BBW-V3" } /***************************************************** Loop function /*****************************************************/ void loop() { Set_Shift(1); //shift display to the right }
Autoscroll text
This code autoscrolls the text "NHD-0216K3Z-NSW-BBW-V3".
/***************************************************** This code was written for the Arduino UNO using SPI Interface. This code displays the part number: NHD-0216K3Z-NSW-BBW-V3 while shifting to the right. /* SPI Wiring Reference for: NHD-0216K3Z-NSW-BBW-V3 --------------------------- LCD | Arduino --------------------------- 1(SPISS) --> 12 2(SDO) --> No Connect 3(SCK) --> 10 4(SDA) --> 11 5(VSS) --> Ground 6(VDD) --> 5V */ /***************************************************** Arduino pin definition /*****************************************************/ #define SPISS_PIN 12 #define SDA_PIN 11 #define SCK_PIN 10 /***************************************************** SPI function to send command and data. For more information on SPI please visit our Support Center /*****************************************************/ void SPI_Out(unsigned char a) { digitalWrite(SPISS_PIN, LOW); for (int n = 0; n < 8; n++) { if ((a & 0x80) == 0x80) { digitalWrite(SDA_PIN, HIGH); delayMicroseconds(200); } else { digitalWrite(SDA_PIN, LOW); } delayMicroseconds(200); a = a << 1; digitalWrite(SCK_PIN, LOW); digitalWrite(SCK_PIN, HIGH); delayMicroseconds(200); } digitalWrite(SCK_PIN, HIGH); delayMicroseconds(200); digitalWrite(SPISS_PIN, HIGH); } /***************************************************** Set Arduino UNO IO ports as Output mode /*****************************************************/ void Set_Pins() { pinMode(SPISS_PIN, OUTPUT); pinMode(SCK_PIN, OUTPUT); pinMode(SDA_PIN, OUTPUT); } /***************************************************** Set LCD Contrast /*****************************************************/ void Set_Contrast() { SPI_Out(0xFE); //prefix command SPI_Out(0x52); //contrast command SPI_Out(0x28); //set contrast value delayMicroseconds(500); } /***************************************************** Set LCD Backlight /*****************************************************/ void Set_Backlight() { SPI_Out(0xFE); //prefix command SPI_Out(0x53); //backlight command SPI_Out(0x08); //set backlight value delayMicroseconds(100); } /***************************************************** Clear LCD /*****************************************************/ void Clear_Display() { SPI_Out(0xFE); //prefix command SPI_Out(0x51); //clear display command delayMicroseconds(1500); } /***************************************************** Set cursor Mode = 0 sets Blinking cursor off Mode = 1 sets Blinking cursor on /*****************************************************/ void Set_Cursor(int mode) { SPI_Out(0xfe); //prefix command if (mode == 0) { SPI_Out(0x4c); //Blinking cursor off } else if (mode == 1) { SPI_Out(0x4b); //Blinking cursor on } delayMicroseconds(100); } /***************************************************** Set Row address Line = 1 sets for first row Line = 2 sets for second row /*****************************************************/ void Set_Row(int line) { SPI_Out(0xfe); //prefix command SPI_Out(0x45); //set cursor command if (line == 1) { SPI_Out(0x00); //cursor position: row one, column one } else if (line == 2) { SPI_Out(0x40); //cursor position: row two, column one } delayMicroseconds(100); } /***************************************************** Write "NHD-0216K3Z-NSW-BBW-V3" to LCD /*****************************************************/ void Part_Number() { Set_Row(1); SPI_Out(0x4e); // N SPI_Out(0x48); // H SPI_Out(0x44); // D SPI_Out(0xb0); // - SPI_Out(0x30); // 0 SPI_Out(0x32); // 2 SPI_Out(0x31); // 1 SPI_Out(0x36); // 6 SPI_Out(0x4b); // K SPI_Out(0x33); // 3 SPI_Out(0x5a); // Z SPI_Out(0xb0); // - SPI_Out(0x4e); // N SPI_Out(0x53); // S SPI_Out(0x57); // W SPI_Out(0xb0); // - Set_Row(2); SPI_Out(0x42); // B SPI_Out(0x42); // B SPI_Out(0x57); // W SPI_Out(0xb0); // - SPI_Out(0x56); // V SPI_Out(0x33); // 3 } /***************************************************** Set Shifting mode Mode = 0 Move display one place to the left Mode = 1 Move display one place to the right delay(500) = 500 Milliseconds /*****************************************************/ void Set_Shift(int mode) { SPI_Out(0xfe); //prefix command if (mode == 0) { SPI_Out(0x55); //Move display one place to the left } else if (mode == 1) { SPI_Out(0x56); //Move display one place to the right } delay(500); } /***************************************************** Main function /*****************************************************/ void setup() { Set_Pins(); //set IO ports Set_Contrast(); //set display contrast Set_Backlight(); //set display backlight Clear_Display(); //clear display Set_Cursor(0); //turns off blinking cursor Part_Number(); //display "NHD-0216K3Z-NSW-BBW-V3" } /***************************************************** Loop function /*****************************************************/ void loop() { Set_Shift(1); //shift display to the right }
Adjusting the LCD contrast
This code displays the text "NHD-0216K3Z-NSW-BBW-V3" while changing the contrast.
/***************************************************** This code was written for the Arduino UNO using SPI Interface. This code displays the part number: NHD-0216K3Z-NSW-BBW-V3 while changing the contrast. /* SPI Wiring Reference for: NHD-0216K3Z-NSW-BBW-V3 --------------------------- LCD | Arduino --------------------------- 1(SPISS) --> 12 2(SDO) --> No Connect 3(SCK) --> 10 4(SDA) --> 11 5(VSS) --> Ground 6(VDD) --> 5V */ /***************************************************** Arduino pin definition /*****************************************************/ #define SPISS_PIN 12 #define SDA_PIN 11 #define SCK_PIN 10 /***************************************************** SPI function to send command and data. For more information on SPI please visit our Support Center /*****************************************************/ void SPI_Out(unsigned char a) { digitalWrite(SPISS_PIN, LOW); for (int n = 0; n < 8; n++) { if ((a & 0x80) == 0x80) { digitalWrite(SDA_PIN, HIGH); delayMicroseconds(200); } else { digitalWrite(SDA_PIN, LOW); } delayMicroseconds(200); a = a << 1; digitalWrite(SCK_PIN, LOW); digitalWrite(SCK_PIN, HIGH); delayMicroseconds(200); } digitalWrite(SCK_PIN, HIGH); delayMicroseconds(200); digitalWrite(SPISS_PIN, HIGH); } /***************************************************** Set Arduino UNO IO ports as Output mode /*****************************************************/ void Set_Pins() { pinMode(SPISS_PIN, OUTPUT); pinMode(SCK_PIN, OUTPUT); pinMode(SDA_PIN, OUTPUT); } /***************************************************** Set LCD Contrast /*****************************************************/ void Set_Contrast() { SPI_Out(0xFE); //prefix command SPI_Out(0x52); //contrast command SPI_Out(0x32); //set contrast value delayMicroseconds(500); } /***************************************************** Set LCD Backlight /*****************************************************/ void Set_Backlight() { SPI_Out(0xFE); //prefix command SPI_Out(0x53); //backlight command SPI_Out(0x08); //set backlight value delayMicroseconds(100); } /***************************************************** Clear LCD /*****************************************************/ void Clear_Display() { SPI_Out(0xFE); //prefix command SPI_Out(0x51); //clear display command delayMicroseconds(1500); } /***************************************************** Set cursor Mode = 0 sets Blinking cursor off Mode = 1 sets Blinking cursor on /*****************************************************/ void Set_Cursor(int mode) { SPI_Out(0xfe); //prefix command if (mode == 0) { SPI_Out(0x4c); //Blinking cursor off } else if (mode == 1) { SPI_Out(0x4b); //Blinking cursor on } delayMicroseconds(100); } /***************************************************** Set Row address Line = 1 sets for first row Line = 2 sets for second row /*****************************************************/ void Set_Row(int line) { SPI_Out(0xfe); //prefix command SPI_Out(0x45); //set cursor command if (line == 1) { SPI_Out(0x00); //cursor position: row one, column one } else if (line == 2) { SPI_Out(0x40); //cursor position: row two, column one } delayMicroseconds(100); } /***************************************************** Write "NHD-0216K3Z-NSW-BBW-V3" to LCD /*****************************************************/ void Part_Number() { Set_Row(1); SPI_Out(0x4e); // N SPI_Out(0x48); // H SPI_Out(0x44); // D SPI_Out(0xb0); // - SPI_Out(0x30); // 0 SPI_Out(0x32); // 2 SPI_Out(0x31); // 1 SPI_Out(0x36); // 6 SPI_Out(0x4b); // K SPI_Out(0x33); // 3 SPI_Out(0x5a); // Z SPI_Out(0xb0); // - SPI_Out(0x4e); // N SPI_Out(0x53); // S SPI_Out(0x57); // W SPI_Out(0xb0); // - Set_Row(2); SPI_Out(0x42); // B SPI_Out(0x42); // B SPI_Out(0x57); // W SPI_Out(0xb0); // - SPI_Out(0x56); // V SPI_Out(0x33); // 3 } /***************************************************** Function to: - change contrast from highest --> lowest - change contrast from lowest --> highest delay(100) = 100 Milliseconds /*****************************************************/ void Contrast_Cycle() { for (int x = 50; x >= 0; x--) { SPI_Out(0xFE); //High to Low contrast loop SPI_Out(0x52); SPI_Out(x); delay(100); } for (int x = 0; x <= 50; x++) { SPI_Out(0xFE); //Low to High contrats loop SPI_Out(0x52); SPI_Out(x); delay(100); } } /***************************************************** Main function /*****************************************************/ void setup() { Set_Pins(); //set IO ports Set_Contrast(); //set display contrast Set_Backlight(); //set display backlight Clear_Display(); //clear display Set_Cursor(0); //turns off blinking cursor Part_Number(); //display "NHD-0216K3Z-NSW-BBW-V3" } /***************************************************** Loop function /*****************************************************/ void loop() { Contrast_Cycle(); //cycle through contrast }
How to use the LCD backlight
There are different methods for controlling or adjusting the backlight of the LCD. Two of the most common ways of controlling or adjusting the LCD backlight are via a command or a potentiometer.
For our serial displays like the NHD-0216K3Z-NSW-BBW-V3, the backlight can be controlled via a command. The
Backlight_loop()
function sets the backlight brightness value from highest to lowest and then from lowest to highest in a constant loop.
The code below displays the text "NHD-0216K3Z-NSW-BBW-V3" while changing the backlight intensity.
/***************************************************** This code was written for the Arduino UNO using SPI Interface. This code displays the part number: NHD-0216K3Z-NSW-BBW-V3 while changing the backlight. /* SPI Wiring Reference for: NHD-0216K3Z-NSW-BBW-V3 --------------------------- LCD | Arduino --------------------------- 1(SPISS) --> 12 2(SDO) --> No Connect 3(SCK) --> 10 4(SDA) --> 11 5(VSS) --> Ground 6(VDD) --> 5V */ /***************************************************** Arduino pin definition /*****************************************************/ #define SPISS_PIN 12 #define SDA_PIN 11 #define SCK_PIN 10 /***************************************************** SPI function to send command and data. For more information on SPI please visit our Support Center /*****************************************************/ void SPI_OUT(unsigned char a) { digitalWrite(SPISS_PIN, LOW); for (int n = 0; n < 8; n++) { if ((a & 0x80) == 0x80) { digitalWrite(SDA_PIN, HIGH); delayMicroseconds(200); } else { digitalWrite(SDA_PIN, LOW); } delayMicroseconds(200); a = a << 1; digitalWrite(SCK_PIN, LOW); digitalWrite(SCK_PIN, HIGH); delayMicroseconds(200); } digitalWrite(SCK_PIN, HIGH); delayMicroseconds(200); digitalWrite(SPISS_PIN, HIGH); } /***************************************************** Set Arduino UNO IO ports as Output mode /*****************************************************/ void Set_Pins() { pinMode(SPISS_PIN, OUTPUT); pinMode(SCK_PIN, OUTPUT); pinMode(SDA_PIN, OUTPUT); } /***************************************************** Set LCD Contrast /*****************************************************/ void Set_Contrast() { SPI_OUT(0xFE); //prefix command SPI_OUT(0x52); //contrast command SPI_OUT(0x28); //set contrast value delayMicroseconds(500); } /***************************************************** Set LCD Backlight /*****************************************************/ void Set_Backlight() { SPI_OUT(0xFE); //prefix command SPI_OUT(0x53); //backlight command SPI_OUT(0x08); //set backlight value delayMicroseconds(100); } /***************************************************** Clear LCD /*****************************************************/ void Clear_Display() { SPI_OUT(0xFE); //prefix command SPI_OUT(0x51); //clear display command delayMicroseconds(1500); } /***************************************************** Set cursor Mode = 0 sets Blinking cursor off Mode = 1 sets Blinking cursor on /*****************************************************/ void Set_Cursor(int mode) { SPI_OUT(0xfe); //prefix command if (mode == 0) { SPI_OUT(0x4c); //Blinking cursor off } else if (mode == 1) { SPI_OUT(0x4b); //Blinking cursor on } delayMicroseconds(100); } /***************************************************** Set Row address Line = 1 sets for first row Line = 2 sets for second row /*****************************************************/ void Set_Row(int line) { SPI_OUT(0xfe); //prefix command SPI_OUT(0x45); //set cursor command if (line == 1) { SPI_OUT(0x00); //cursor position: row one, column one } else if (line == 2) { SPI_OUT(0x40); //cursor position: row two, column one } delayMicroseconds(100); } /***************************************************** Write "NHD-0216K3Z-NSW-BBW-V3" to LCD /*****************************************************/ void Part_Number() { Set_Row(1); SPI_OUT(0x4e); // N SPI_OUT(0x48); // H SPI_OUT(0x44); // D SPI_OUT(0xb0); // - SPI_OUT(0x30); // 0 SPI_OUT(0x32); // 2 SPI_OUT(0x31); // 1 SPI_OUT(0x36); // 6 SPI_OUT(0x4b); // K SPI_OUT(0x33); // 3 SPI_OUT(0x5a); // Z SPI_OUT(0xb0); // - SPI_OUT(0x4e); // N SPI_OUT(0x53); // S SPI_OUT(0x57); // W SPI_OUT(0xb0); // - Set_Row(2); SPI_OUT(0x42); // B SPI_OUT(0x42); // B SPI_OUT(0x57); // W SPI_OUT(0xb0); // - SPI_OUT(0x56); // V SPI_OUT(0x33); // 3 } /***************************************************** Function to: - change backlight from highest --> lowest - change backlight from lowest --> highest delay(500) = 500 Milliseconds /*****************************************************/ void Backlight_loop() { for (int x = 8; x >= 0; x--) { SPI_OUT(0xFE); //High to Low Backlight loop SPI_OUT(0x53); SPI_OUT(x); delay(500); } for (int x = 0; x <= 8; x++) { SPI_OUT(0xFE); //Low to High Backlight loop SPI_OUT(0x53); SPI_OUT(x); delay(500); } } /***************************************************** Main function /*****************************************************/ void setup() { Set_Pins(); //set IO ports Set_Contrast(); //set display contrast Set_Backlight(); //set display backlight Clear_Display(); //clear display Set_Cursor(0); //turns off blinking cursor Part_Number(); //display "NHD-0216K3Z-NSW-BBW-V3" } /***************************************************** Loop function /*****************************************************/ void loop() { Backlight_loop(); //High to Low Backlight loop }
Characters/font tables
This code displays characters from the font table that is included in the LCD.
/***************************************************** This code was written for the Arduino UNO using SPI Interface. This code displays the font table characters. /* SPI Wiring Reference for: NHD-0216K3Z-NSW-BBW-V3 --------------------------- LCD | Arduino --------------------------- 1(SPISS) --> 12 2(SDO) --> No Connect 3(SCK) --> 10 4(SDA) --> 11 5(VSS) --> Ground 6(VDD) --> 5V */ /***************************************************** Arduino pin definition /*****************************************************/ #define SPISS_PIN 12 #define SDA_PIN 11 #define SCK_PIN 10 /***************************************************** SPI function to send command and data. For more information on SPI please visit our Support Center /*****************************************************/ void SPI_out(unsigned char a) { digitalWrite(SPISS_PIN, LOW); for (int n = 0; n < 8; n++) { if ((a & 0x80) == 0x80) { digitalWrite(SDA_PIN, HIGH); delayMicroseconds(200); } else { digitalWrite(SDA_PIN, LOW); } delayMicroseconds(200); a = a << 1; digitalWrite(SCK_PIN, LOW); digitalWrite(SCK_PIN, HIGH); delayMicroseconds(200); } digitalWrite(SCK_PIN, HIGH); delayMicroseconds(200); digitalWrite(SPISS_PIN, HIGH); } /***************************************************** Set Arduino UNO IO ports as Output mode /*****************************************************/ void Set_Pins() { pinMode(SPISS_PIN, OUTPUT); pinMode(SCK_PIN, OUTPUT); pinMode(SDA_PIN, OUTPUT); } /***************************************************** Set LCD Contrast /*****************************************************/ void Set_Contrast() { SPI_out(0xFE); //prefix command SPI_out(0x52); //contrast command SPI_out(0x28); //set contrast value delayMicroseconds(500); } /***************************************************** Set LCD Backlight /*****************************************************/ void Set_Backlight() { SPI_out(0xFE); //prefix command SPI_out(0x53); //backlight command SPI_out(0x08); //set backlight value delayMicroseconds(100); } /***************************************************** Clear LCD /*****************************************************/ void Clear_Display() { SPI_out(0xFE); //prefix command SPI_out(0x51); //clear display command delayMicroseconds(1500); } /***************************************************** Set cursor Mode = 0 sets Blinking cursor off Mode = 1 sets Blinking cursor on /*****************************************************/ void Set_Cursor(int mode) { SPI_out(0xfe); //prefix command if (mode == 0) { SPI_out(0x4c); //Blinking cursor off } else if (mode == 1) { SPI_out(0x4b); //Blinking cursor on } delayMicroseconds(100); } /***************************************************** Set Row address Line = 1 sets for first row Line = 2 sets for second row /*****************************************************/ void Set_Row(int line) { SPI_out(0xfe); //prefix command SPI_out(0x45); //set cursor command if (line == 1) { SPI_out(0x00); //cursor position: row one, column one } else if (line == 2) { SPI_out(0x40); //cursor position: row two, column one } delayMicroseconds(100); } /***************************************************** Display font characters -change the row address every 16 characters displayed -used Clear_Display() function to clear the display and change the row to the first row delay(500) = 500 Milliseconds /*****************************************************/ void Font_Table_Characters() { Clear_Display(); for (int i = 0; i < 64; i++) { if (i == 16 || i == 48) { Set_Row(2); } else if (i == 32 || i == 64) { Clear_Display(); if (i == 64) { i = 0; } } SPI_out(i + 32); delay(500); } } /***************************************************** Main function /*****************************************************/ void setup() { Set_Pins(); //set IO ports Set_Contrast(); //set display contrast Set_Backlight(); //set display backlight Clear_Display(); //clear display Set_Cursor(0); //turns off blinking cursor } /***************************************************** Loop function /*****************************************************/ void loop() { Font_Table_Characters(); //Display font characters }
Circuit - Connection Diagram and Schematic for RS232 TTL Communication
RS232 TTL is a serial communication protocol that uses RS232-type specs but with TTL logic levels (0V to Vcc, typically 3.3V or 5V). The RS232 TTL connection circuit between Arduino and the 16-character Newhaven display uses only three wires:
Arduino | LCD | Connection Type |
---|---|---|
5V Pin | Pin 3: V DD | Power |
Ground Pin | Pin 2: Ground | Ground |
Pin 7: Digital I/O pin | Pin 1: RX | RS-232 (TTL) Serial input port |
Note:
R1 and R2 on the display must be open to enable RS232 TTL as specified in the
datasheet.
RS232 TTL Wiring circuit
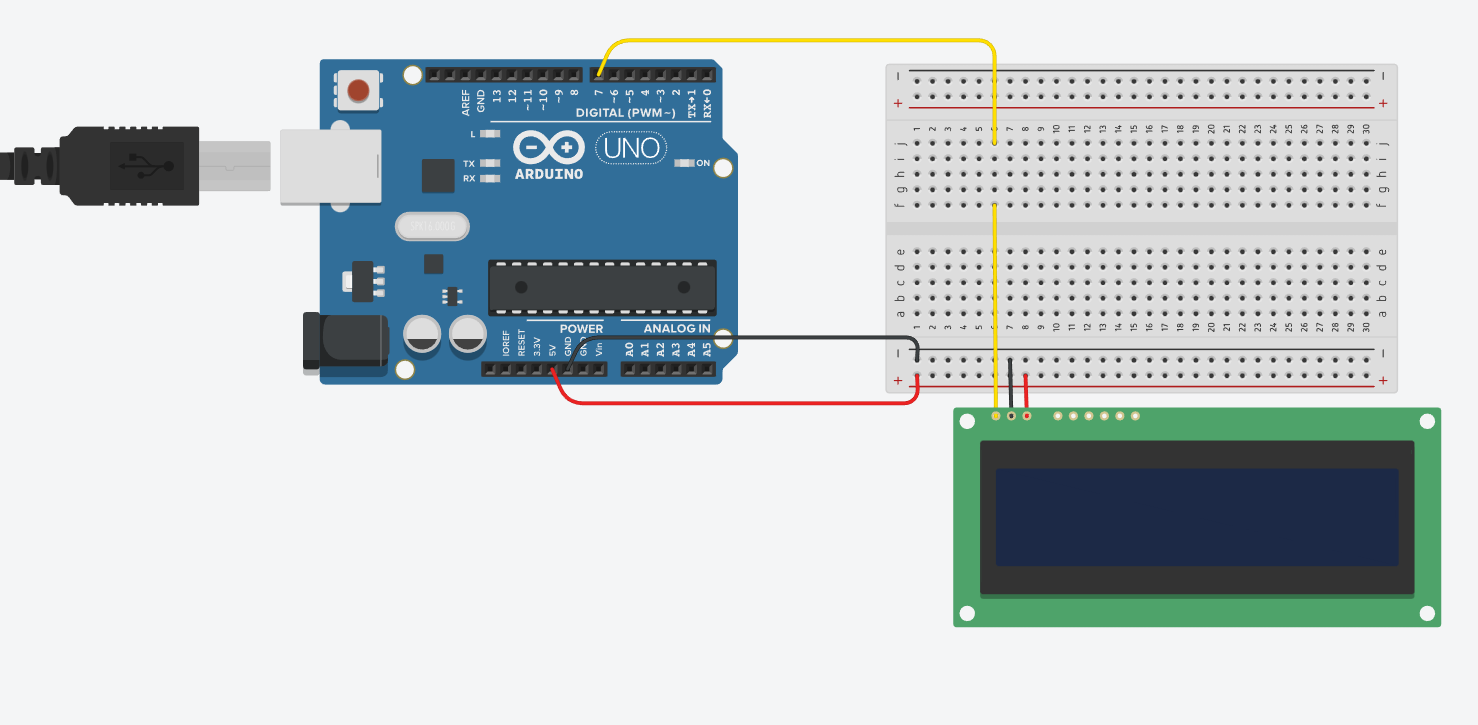
RS232 TTL Wiring Schematic
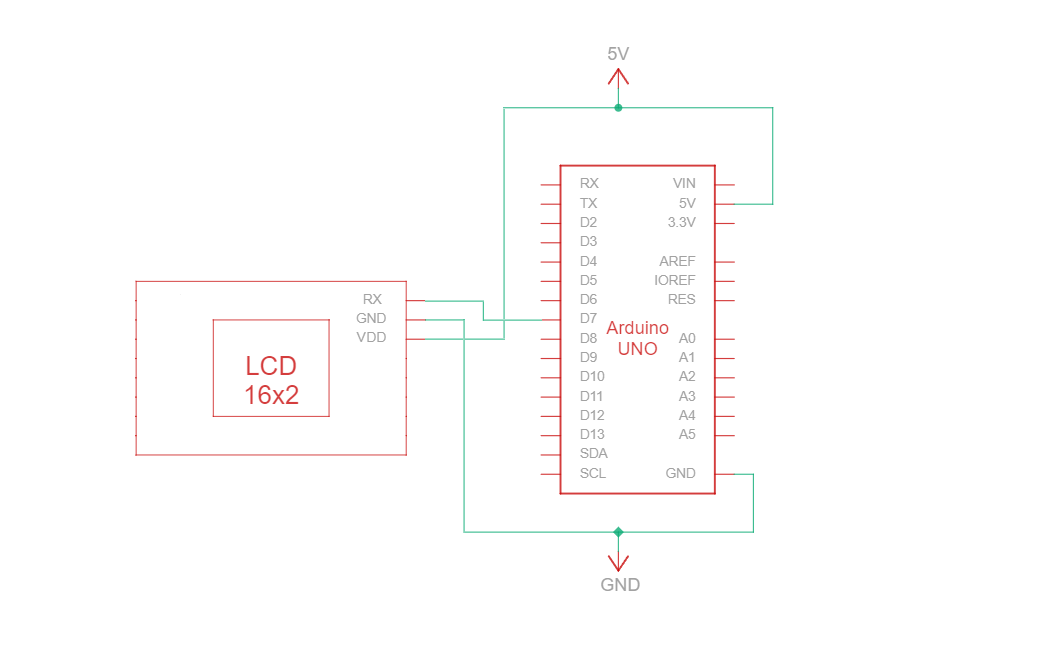
We have created a detailed beginner-friendly tutorial on Instructables for RS232 TTL communication. The tutorial includes all the necessary steps to complete this project. Check it out here: www.instructables.com/Arduino-LCD-Tutorial/
Arduino - LCD Hello World! Code Example Using serial RS232 TTL communication
The following code example displays "Hello Word!" on the LCD using RS232 TTL communication.
/***************************************************** This code was written for the Arduino UNO using RS-232 Interface. /* RS-232 Wiring Reference for: NHD-0216K3Z-NSW-BBW-V3 --------------------------- LCD | Arduino --------------------------- 1(RX) --> 7 2(VSS) --> Ground 3(VDD) --> 5V */ #define P1 7 //RX the pin on which to receive serial data. /***************************************************** RS-232 setup for command and data. For more information on RS-232 please visit our Support Center /*****************************************************/ #include <SoftwareSerial.h> #define RxPin P1 SoftwareSerial NHD_LCD = SoftwareSerial(P1, RxPin); /***************************************************** Set LCD Contrast /*****************************************************/ void Set_Contrast() { NHD_LCD.write(0xFE); //Prefix command NHD_LCD.write(0x52); //Contrast command NHD_LCD.write(0x28); //Set contrast value delayMicroseconds(500); } /***************************************************** Clear LCD /*****************************************************/ void Clear_display() { NHD_LCD.write(0xFE); //Prefix command NHD_LCD.write(0x51); //Clear Display delayMicroseconds(1500); } /***************************************************** Set Arduino UNO IO ports as Output mode /*****************************************************/ void Set_Pins() { pinMode(RxPin, OUTPUT); } /***************************************************** Set LCD Backlight /*****************************************************/ void Set_Backlight() { NHD_LCD.write(0xFE); //prefix command NHD_LCD.write(0x53); //Backlight command NHD_LCD.write(0x08); //Set backlight value delayMicroseconds(100); } /***************************************************** Set cursor Mode = 0 sets Blinking cursor off Mode = 1 sets Blinking cursor on /*****************************************************/ void Set_Cursor(int mode) { NHD_LCD.write(0xFE); //Prefix command if (mode == 0) { NHD_LCD.write(0x4c); //Turn blinking cursor off } else if (mode == 1) { NHD_LCD.write(0x4b); //Turn blinking cursor on } delayMicroseconds(100); } /***************************************************** Write "Hello World!" to LCD /*****************************************************/ void Hello_World() { NHD_LCD.write(0x48); // H NHD_LCD.write(0x65); // e NHD_LCD.write(0x6C); // l NHD_LCD.write(0x6C); // l NHD_LCD.write(0x6F); // o NHD_LCD.write(0x11); // Blank NHD_LCD.write(0x57); // W NHD_LCD.write(0x6f); // o NHD_LCD.write(0x72); // r NHD_LCD.write(0x6c); // l NHD_LCD.write(0x64); // d NHD_LCD.write(0x21); // ! } /***************************************************** Main function /*****************************************************/ void setup() { NHD_LCD.begin(9600); //Sets 9600 Baud Rate the display can receive and transmit data at a rate of 9600 bits per second by default. delay(15); Set_Pins(); //Set IO ports Set_Cursor(0); //Turns off blinking cursor Set_Contrast(); //Set display Contrast Set_Backlight(); //Set display Backlight Clear_display(); //Clear display Hello_World(); //Display "Hello Wolrd!" } void loop() { }
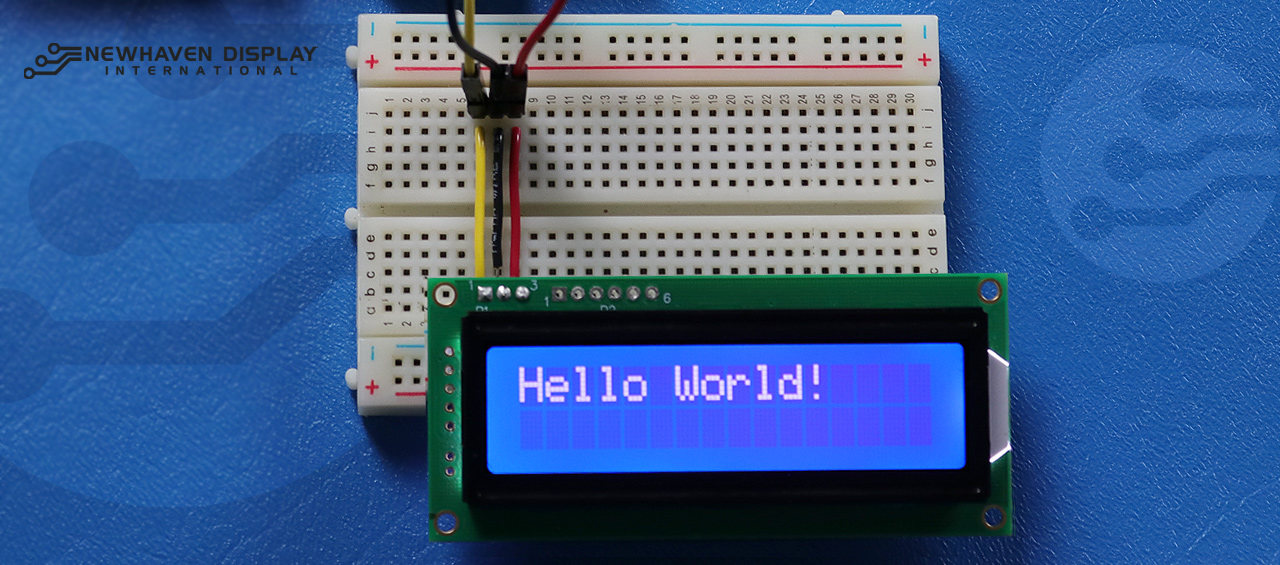
Troubleshooting Your LCD - Arduino Project
Below we will discuss some common steps to take when encountering issues or problems with your Arduino - LCD project.
- Check wiring connections: The most common issue when connecting an LCD to an Arduino is incorrect wiring. Check your wiring connections against the provided pinout diagram and circuit to ensure proper connectivity. Additionally, keep in mind that the NHD-0216K3Z-NSW-BBW-V3 display can be configured to work with SPI, I2C, and RS232 TTL interfaces. A 0 ohm resistor must be soldered onto the R2 section in the PCB to work with the SPI interface.
- Inspect components: Look for any visible signs of damage on the Arduino, LCD, or connecting wires.
- Verify the code: Ensure the uploaded code on your Arduino is correct and free from errors.
- Check the power supply: Ensure that your power source is sufficient to power both the Arduino and the LCD.
- Test with a known-good LCD: If you are still experiencing issues, try connecting a different, known-good LCD to your Arduino. This will help you determine if the problem is with the LCD or the Arduino board itself.
- Adjust contrast: If you are having trouble seeing the displayed text on the LCD, try adjusting the contrast using the software function
Set_Contrast()
, which is described above. Different lighting conditions might require different contrast settings for optimal visibility. - Check the Arduino IDE version: Ensure that you are using the latest version the Arduino IDE. Outdated versions might contain bugs or be incompatible with your project.
- Test individual features: If you are having issues with specific features like scrolling or contrast control, try running simpler code like the "Hello World!" example to isolate the problem. This can help you determine if the issue is related to the code or the hardware.
- Seek help from the community: If you are unable to resolve the issue after following the above troubleshooting steps, consider seeking help from the online community. Online forums, such as the Newhaven Display Support Forum, the Arduino Stack Exchange, or the Arduino.cc forum, are excellent resources for getting assistance from experienced users.
By following these troubleshooting steps, you should be able to diagnose and resolve most issues related to connecting an LCD to an Arduino board. Remember, patience and attention to detail are key when troubleshooting electronics projects.
Additional Resources
- Arduino UNO official website.
- LCD 16x2 product description.
- LCD 16x2 product datasheet and specs.
- Beginner-friendly Arduino-LCD Instructables Tutorial
- Character LCD Support Forum.
- Read our beginner's guide to prototyping displays.
- Character LCD sample code.
- Learn how to protect electronics from ESD.
- Learn about the types of LCDs.
- Learn about LCD modes.
Latest Blog Posts
-
Passive Matrix vs Active Matrix - A Beginner's Guide
Have you ever wondered how display screens are able to produce sharp images and vibrant colors? T …May 30th 2024 -
How to Display Images on a TFT LCD
TFT LCDs, or thin-film transistor liquid-crystal displays, are a type of LCD commonly used in var …Apr 2nd 2024 -
Brightness Enhancement Film (BEF)
Over the years, display manufacturers have been developing new technologies to improve image qual …Mar 6th 2024